SaaSHub helps you find the best software and product alternatives Learn more β
Top 23 Rust Markdown Projects
-
gutenberg
A fast static site generator in a single binary with everything built-in. https://www.getzola.org
-
WorkOS
The modern identity platform for B2B SaaS. The APIs are flexible and easy-to-use, supporting authentication, user identity, and complex enterprise features like SSO and SCIM provisioning.
-
InfluxDB
Power Real-Time Data Analytics at Scale. Get real-time insights from all types of time series data with InfluxDB. Ingest, query, and analyze billions of data points in real-time with unbounded cardinality.
-
tp-note
Minimalistic note taking: save and edit your clipboard content as a note file (Gitlab mirror)
-
orion
A static site generator written in Rust to create a simple blog from Markdown files (by adriantombu)
-
SaaSHub
SaaSHub - Software Alternatives and Reviews. SaaSHub helps you find the best software and product alternatives
So after shopping around a bit I found a simple, dependency-less static site generator called Zola. The lack of dependencies sounded very attractive after all the headaches trying to update my Gatsby modules. I wanted to give Zola a try and see what tradeoffs I would need to make coming form a React-based framework to this Rust-based generator.
Project mention: Oxlint β written in Rust β 50-100 Times Faster than ESLint | news.ycombinator.com | 2023-12-15
Project mention: CryptoFlow: Building a secure and scalable system with Axum and SvelteKit - Part 3 | dev.to | 2024-01-08As a platform that allows expressiveness, we want our users to be bold enough to ask and answer questions with either plain text or some markdowns. Compiling markdown to HTML in Rust can be done via the pulldown-cmark crate. We used it in this utility function:
Project mention: Ubiquity (v0.3.0) - I made a cross-platform markdown editor to learn some Rust. It uses Yew, Tauri, Tailwind and DaisyUI. Currently available on Windows, Linux and the web. | /r/rust | 2023-07-10I used the markdown-rs crate for Ubiquity.
I use the Markdown Composer for many years now. Itβs fast and it simply opens a tab in your browser.
I am developer of the note-talking tool Tp-Note. It uses the file system to store notes, with prepended tags, e.g. 20230515-French Fries--howto.md. So far, the Tp-Note documentation refers to the tag 20230515 as "sort-tag" (= Sortierkennzeichen in German). It seems my translation is not right. Is the term order tag more appropriate? There are 2 variants: chronological sort/order tag and sequential sort/order tag. Are you English native? Please comment which term is more accurate. Or, is there a better word?
It's also gained a website hosting documentation.
Project mention: RSS can be used to distribute all sorts of information | news.ycombinator.com | 2023-11-20Just a GitHub. [1]
It's very early days still, but I use it myself.
The marketplace [2] is much more advanced! You can already use it to buy and sell stuff over Nostr!
[1] https://github.com/servuscms/servus
Rust Markdown related posts
- TSDocs.dev: type docs for any JavaScript library
- Ubiquity (v0.3.0) - I made a cross-platform markdown editor to learn some Rust. It uses Yew, Tauri, Tailwind and DaisyUI. Currently available on Windows, Linux and the web.
- MdBook β Create book from Markdown files. Like Gitbook but implemented in Rust
- Any alternatives to Obsidian that are not built on Electron?
- Tagging note files: `order tag` vs `sort tag`
- Project idea: port markdownlint to Rust
- Tp-Note: Minimalistic note taking
-
A note from our sponsor - SaaSHub
www.saashub.com | 26 Apr 2024
Index
What are some of the best open-source Markdown projects in Rust? This list will help you:
Project | Stars | |
---|---|---|
1 | gutenberg | 12,673 |
2 | dprint | 2,933 |
3 | pulldown-cmark | 1,923 |
4 | comrak | 1,074 |
5 | termimad | 840 |
6 | markdown-rs | 765 |
7 | crowbook | 687 |
8 | vim-markdown-composer | 618 |
9 | fastn | 463 |
10 | obsidian-extract-url | 229 |
11 | rust-book-fr | 159 |
12 | cbfmt | 158 |
13 | tp-note | 113 |
14 | marko-editor | 60 |
15 | bard | 44 |
16 | servus | 40 |
17 | gisture | 30 |
18 | criterion-table | 22 |
19 | mini_markdown | 18 |
20 | makurust | 17 |
21 | parsedown | 16 |
22 | orion | 14 |
23 | tax | 14 |
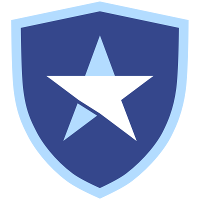
Sponsored