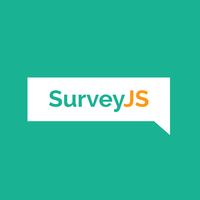
-
styled-components
Visual primitives for the component age. Use the best bits of ES6 and CSS to style your apps without stress đź’…
-
SurveyJS
Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App. With SurveyJS form UI libraries, you can build and style forms in a fully-integrated drag & drop form builder, render them in your JS app, and store form submission data in any backend, inc. PHP, ASP.NET Core, and Node.js.
function App() { return ( Your Name: Your Age: 0 - 17 18 - 80 80 + If you love React, check this box => Comments: Submit ) } export default App; const formStyle = () => { return { display:"flex", flexDirection:"column", margin:"40px auto", lineHeight: "50px", width: "400px", fontSize:"20px" } }
Enter fullscreen mode Exit fullscreen mode(I've included a formStyle() function that's used for inline styling for readability purposes in your browser. There are much better ways to style components FYI.)
Right now, this form is considered uncontrolled, and anything you type or select lives in your browser’s DOM.
To make this a Control Form, we need to incorporate state into our input, select, and textarea tags. To start, we need to add state to our component.
1. Add State
On line 1 in App.js, import the useState hook.
import { useState } from 'react';
Then, inside the App component & before the return statement (line 4), let's declare our state variables.
const [name, setName] = useState(""); const [age, setAge] = useState("young"); const [opinion, setOpinion] = useState("false"); const [comments, setComments] = useState("");
Enter fullscreen mode Exit fullscreen modeWe've destructured the useState hook for each variable, each having its own state variable set to the initial value declared in useState(), and a setter function we'll use to update state every time the user interacts with the form.
2. Assign State
Next, let's add state variables to our form elements. In these elements, add the 'value' attribute and assign their state variable.
... ... ... </code></pre> <div class="highlight__panel js-actions-panel"> <div class="highlight__panel-action js-fullscreen-code-action"> <svg xmlns="http://www.w3.org/2000/svg" width="20px" height="20px" viewbox="0 0 24 24" class="highlight-action crayons-icon highlight-action--fullscreen-on"><title>Enter fullscreen mode</title> <path d="M16 3h6v6h-2V5h-4V3zM2 3h6v2H4v4H2V3zm18 16v-4h2v6h-6v-2h4zM4 19h4v2H2v-6h2v4z"></path> </svg> <svg xmlns="http://www.w3.org/2000/svg" width="20px" height="20px" viewbox="0 0 24 24" class="highlight-action crayons-icon highlight-action--fullscreen-off"><title>Exit fullscreen mode</title> <path d="M18 7h4v2h-6V3h2v4zM8 9H2V7h4V3h2v6zm10 8v4h-2v-6h6v2h-4zM8 15v6H6v-4H2v-2h6z"></path> </svg> </div> </div> </div> <p>At this point, if you try to interact with our form in your DOM you'll notice each field is frozen. If you look in your browser's console, you’ll see an error message:<br> <code>Warning: You provided a 'value' prop to a form field without an 'onChange' handler...</code><br> Have no fear. This is because we haven't added our setters to an event listener yet.</p> <h2> <a name="3-listen-for-state-changes" href="#3-listen-for-state-changes"> </a> 3. Listen for State Changes </h2> <p>Let's add event listeners to our form elements! The onChange event listener in React inputs expects a callback function, and has access to the event object, just like in vanilla JavaScript. </p> <p>We can use event.target.value for input, select, and textarea tags. Radio buttons and checkboxes are a little different, since they're triggered by a boolean value, and can be accessed with event.target.checked. Here's what that looks like.<br> </p> <div class="highlight js-code-highlight"> <pre class="highlight plaintext"><code><input onChange={(e) => setName(e.target.value)} value={name} type="text" /> ... <select onChange={(e) => setAge(e.target.value)} value={age}> ... <input onChange={(e) => setOpinion(e.target.checked)} value={opinion} type="checkbox" /> ... <textarea onChange={(e) => setComments(e.target.value)} value={comments} /> </code></pre> <div class="highlight__panel js-actions-panel"> <div class="highlight__panel-action js-fullscreen-code-action"> <svg xmlns="http://www.w3.org/2000/svg" width="20px" height="20px" viewbox="0 0 24 24" class="highlight-action crayons-icon highlight-action--fullscreen-on"><title>Enter fullscreen mode</title> <path d="M16 3h6v6h-2V5h-4V3zM2 3h6v2H4v4H2V3zm18 16v-4h2v6h-6v-2h4zM4 19h4v2H2v-6h2v4z"></path> </svg> <svg xmlns="http://www.w3.org/2000/svg" width="20px" height="20px" viewbox="0 0 24 24" class="highlight-action crayons-icon highlight-action--fullscreen-off"><title>Exit fullscreen mode</title> <path d="M18 7h4v2h-6V3h2v4zM8 9H2V7h4V3h2v6zm10 8v4h-2v-6h6v2h-4zM8 15v6H6v-4H2v-2h6z"></path> </svg> </div> </div> </div> <p>That's it! Now we have a simple <a href="https://reactjs.org/docs/forms.html#controlled-components">control form</a>w. If you want to test out what we've done so far, inject our state variables into the JSX! Here's our final version of App.js, for your reference.<br> </p> <div class="highlight js-code-highlight"> <pre class="highlight plaintext"><code>import { useState } from 'react'; function App() { const [name, setName] = useState(""); const [age, setAge] = useState("young"); const [opinion, setOpinion] = useState("false"); const [comments, setComments] = useState(""); return ( <> <form style={formStyle()}> <label>Your Name:</label> <input onChange={(e) => setName(e.target.value)} value={name} type="text" /> <label>Your Age:</label> <select onChange={(e) => setAge(e.target.value)} value={age}> <option value="youth">0 - 17</option> <option value="adult">18 - 80</option> <option value="elder">80 +</option> </select> <label> If you love React, check this box => <input onChange={(e) => setOpinion(e.target.checked)} value={opinion} type="checkbox" /> </label> <label>Comments:</label> <textarea onChange={(e) => setComments(e.target.value)} value={comments} /> <button type="submit">Submit</button> </form> <h3>User Name: {name}</h3> <h3>User Age: {age}</h3> <h3>User Opinion: {opinion}</h3> <h3>User Textarea: {comments}</h3> </> ); } export default App; const formStyle = () => { return { display:"flex", flexDirection:"column", margin:"40px auto", lineHeight: "50px", width: "400px", fontSize:"20px" } } </code></pre> <div class="highlight__panel js-actions-panel"> <div class="highlight__panel-action js-fullscreen-code-action"> <svg xmlns="http://www.w3.org/2000/svg" width="20px" height="20px" viewbox="0 0 24 24" class="highlight-action crayons-icon highlight-action--fullscreen-on"><title>Enter fullscreen mode</title> <path d="M16 3h6v6h-2V5h-4V3zM2 3h6v2H4v4H2V3zm18 16v-4h2v6h-6v-2h4zM4 19h4v2H2v-6h2v4z"></path> </svg> <svg xmlns="http://www.w3.org/2000/svg" width="20px" height="20px" viewbox="0 0 24 24" class="highlight-action crayons-icon highlight-action--fullscreen-off"><title>Exit fullscreen mode</title> <path d="M18 7h4v2h-6V3h2v4zM8 9H2V7h4V3h2v6zm10 8v4h-2v-6h6v2h-4zM8 15v6H6v-4H2v-2h6z"></path> </svg> </div> </div> </div>
Let’s see how to make this happen. If you’d like to follow along, make sure you have a code editor of your choice, such as VS Code. Also make sure you have npm and Google Chrome installed, and create a new React app. This might take a few minutes.
Let’s see how to make this happen. If you’d like to follow along, make sure you have a code editor of your choice, such as VS Code. Also make sure you have npm and Google Chrome installed, and create a new React app. This might take a few minutes.