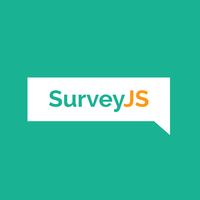
-
sanity-next-stripe-starter
Barebones blog set-up with NextJS and Sanity. Comes with Sanity's inbuilt image handler.
-
SurveyJS
Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App. With SurveyJS form UI libraries, you can build and style forms in a fully-integrated drag & drop form builder, render them in your JS app, and store form submission data in any backend, inc. PHP, ASP.NET Core, and Node.js.
-
InfluxDB
Power Real-Time Data Analytics at Scale. Get real-time insights from all types of time series data with InfluxDB. Ingest, query, and analyze billions of data points in real-time with unbounded cardinality.
git clone[https://github.com/bathrobe/sanity-next-stripe-starter](https://github.com/bathrobe/sanity-next-stripe-starter)
// pages/api/checkout_sessions/cart.js import { validateCartItems } from "use-shopping-cart/src/serverUtil"; import Stripe from "stripe"; import { client } from "../../../lib/sanity/client"; import { merchQuery } from "../../../lib/sanity/merchQuery"; const stripe = new Stripe(process.env.STRIPE_SECRET_KEY, { // https://github.com/stripe/stripe-node#configuration apiVersion: "2020-03-02", }); export default async function handler(req, res) { if (req.method === "POST") { try { // Validate the cart details that were sent from the client. const cartItems = req.body; //Sanity client performs merchQuery let sanityData = await client.fetch(merchQuery); // The POST request is then validated against the data from Sanity. const line_items = validateCartItems(sanityData, cartItems); // Create Checkout Sessions from body params. const params = { submit_type: "pay", mode: "payment", payment_method_types: ["card"], billing_address_collection: "auto", shipping_address_collection: { allowed_countries: ["US", "CA"], }, //The validated cart items are inserted. line_items, success_url: `${req.headers.origin}/result?session_id={CHECKOUT_SESSION_ID}`, cancel_url: `${req.headers.origin}`, }; const checkoutSession = await stripe.checkout.sessions.create(params); res.status(200).json(checkoutSession); } catch (err) { res.status(500).json({ statusCode: 500, message: err.message }); } } else { res.setHeader("Allow", "POST"); res.status(405).end("Method Not Allowed"); } }
Automatically send a confirmation email from the result.js page using a service like Mailgun
We'll be using Vercel's SWR library for fetching, as well as the excellent [use-shopping-cart](https://useshoppingcart.com/) React integration.
Use the Stripe Elements of react-stripe-js to display all checkout UI within your own frontend
Stripe describes itself as "the payments infrastructure of the internet," and is generally recognized as the gold standard of commerce platforms online. Next.js is a fast, popular framework for ReactJS. Sanity, our cutting-edge content platform, is designed to integrate seamlessly with tools like these.
// components/CartSummary.js import { useState, useEffect } from "react"; import { useShoppingCart } from "use-shopping-cart"; import { fetchPostJSON } from "../utils/apiHelpers"; export default function CartSummary() { const [loading, setLoading] = useState(false); const [cartEmpty, setCartEmpty] = useState(true); const { formattedTotalPrice, cartCount, clearCart, cartDetails, redirectToCheckout, } = useShoppingCart(); useEffect(() => setCartEmpty(!cartCount), [cartCount]); const handleCheckout = async (event) => { event.preventDefault(); setLoading(true); const response = await fetchPostJSON( "/api/checkout_sessions/cart", cartDetails ); if (response.statusCode === 500) { console.error(response.message); return; } redirectToCheckout({ sessionId: response.id }); }; return (
Cart summaryh2> {/* This is where we'll render our cart; The item count changes quickly and may be mismatched between client and server. To avoid annoying error messages, we use 'supressHydrationWarning'. https://reactjs.org/docs/dom-elements.html#suppresshydrationwarning*/}
Number of Items:strong> {cartCount} p>
Total:strong> {formattedTotalPrice} p>
Use 4242 4242 4242 4242 as the card number.p> Checkout{" "}
div> button>Clear Cart button> form> ); }
// web/pages/result.js import { useRouter } from "next/router"; import Link from "next/link"; import useSWR from "swr"; import PrintObject from "../components/PrintObject"; import { fetchGetJSON } from "../utils/apiHelpers"; const ResultPage = () => { const router = useRouter(); // Fetch CheckoutSession from static page via // https://nextjs.org/docs/basic-features/data-fetching#static-generation const { data, error } = useSWR( router.query.session_id ? `/api/checkout_sessions/${router.query.session_id}` : null, fetchGetJSON ); if (error) { return
failed to loaddiv>; } return (CongratsCheckout Payment Resulth1>
With the data below, you can display a custom confirmation message to your customer. p>
For example:p>
Thank you, {data?.payment_intent.charges.data[0].billing_details.name}. h3>
Confirmation email sent to{" "} {data?.payment_intent.charges.data[0].billing_details.email}. p>
Status: {data?.payment_intent?.status ?? "loading..."}
CheckoutSession response:h3> Back homea> div> ); }; export default ResultPage;